How to Sync data from one folder to another
- tinyytopic.com
- 0
- on Feb 27, 2023
How to sync data from one folder to another using Python ready-to-use function?
This Python function synchronizes the contents of one folder to another using the robocopy
command. Ready-to-use Python function to sync data from one folder to another:
def sync_folders(source_folder, dest_folder): # Synchronizes folder contents using robocopy cmd = ['robocopy', source_folder, dest_folder, '/e', '/mir', '/np', '/tee', '/mt:4', '/r:1', '/w:5'] with subprocess.Popen(cmd, stdout=subprocess.PIPE, stderr=subprocess.PIPE, universal_newlines=True) as process: for line in process.stdout: print(line, end='') for line in process.stderr: print(line, end='')
Write your main code as a sample below,
import subprocess
sync_folders('C:\Downloads\Data', 'C:\Downloads\Save To')
The output of the code is,
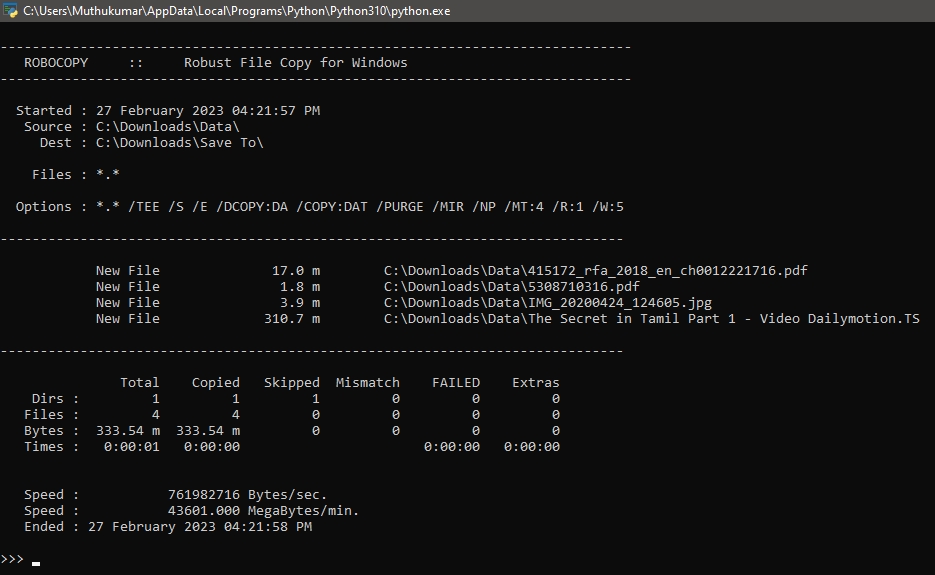
How does the function work?
This is a Python function called sync_folders
that synchronizes the contents of two folders using the robocopy
command
The function takes two arguments, source_folder
and dest_folder
, which are the paths to the source and destination folders, respectively.
Inside the function, a list called cmd
is created, which contains the arguments to pass to the robocopy
command. These arguments include the /e
, /mir
, /np
, /tee
, /mt:4
, /r:1
, and /w:5
options, which instruct robocopy
to copy all subdirectories, mirror the source folder to the destination folder, display progress without a percentage, output to console and log file, use 4 threads, retry once on failed copies, and wait 5 seconds between retries, respectively.
The subprocess.Popen
function is then used to create a new process object, which runs the robocopy
command with the specified arguments. The stdout
, stderr
, and universal_newlines
parameters are set to capture the output of robocopy
as text.
The function then loops through the stdout
and stderr
output using a for
loop, and prints each line to the console using print(line, end='')
.
If there is an error during the execution of the robocopy
command, the error message will be printed to the console as well.